Empowering Your Software Development with Data Annotations
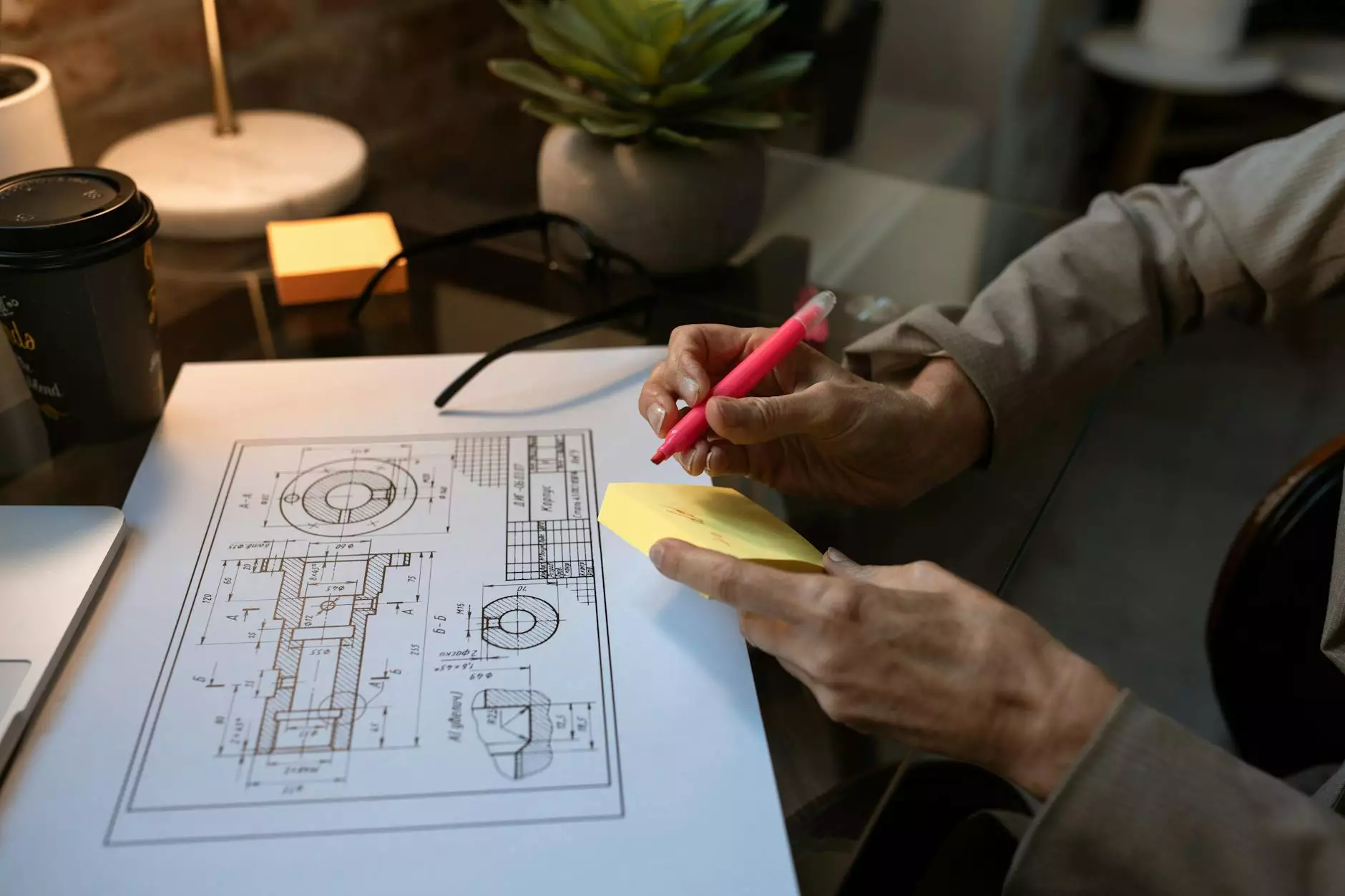
Understanding the Basics of Data Annotations
Data annotations are a powerful concept in the realm of software development, particularly in data management and validation frameworks. They serve as a means to provide metadata to data models, which can be pivotal for ensuring data integrity and enhancing application behavior. Typically associated with ASP.NET MVC and Entity Framework, these attributes allow developers to add rules and constraints to their data models directly, simplifying the validation process.
Why Use Data Annotations in Software Development?
In the fast-paced world of software development, leveraging efficiency is paramount. Using data annotations brings several significant advantages:
- Simplicity: Incorporating annotations directly within model classes reduces the amount of redundant code, making models easier to maintain.
- Validation: Built-in validation attributes ensure that data meets specific criteria before being processed or stored.
- Readable Code: Annotated models enhance code readability and reduce the cognitive load for future developers.
- Integrated Documentation: Annotations act as a form of documentation, indicating constraints and requirements right at the point of data entry.
How Data Annotations Work
Data annotations utilize attributes to specify rules for model properties. Common attributes include:
- [Required]: Ensures that a property must have a value.
- [StringLength(maximumLength, MinimumLength)]: Specifies the maximum and minimum length of string fields.
- [Range(min, max)]: Sets a range of valid values for numeric fields.
- [EmailAddress]: Validates the format of email addresses.
- [RegularExpression(pattern)]: Validates string properties against a specified pattern.
Implementing Data Annotations in Your Application
To effectively implement data annotations, follow these steps:
- Define Your Model: Start by creating a model class to represent your data.
- Apply Annotations: Use the appropriate data annotations for each property of your model class. For instance: public class User { [Required] public string Name { get; set; } [EmailAddress] public string Email { get; set; } [Range(18, 100)] public int Age { get; set; } }
- Validate Your Model: In your application logic, validate the model using the validation mechanism provided by your framework.
- Handle Validation Errors: Ensure that errors are properly handled, providing clear feedback to users.
Best Practices for Using Data Annotations
To maximize the effectiveness of data annotations, consider the following best practices:
- Keep It Consistent: Use data annotations consistently across your application to enforce uniformity in validation rules.
- Limit Custom Logic: Reserve custom validation logic for cases that cannot be addressed by built-in attributes.
- Document Annotations: Clearly document the purpose of each annotation to aid team members who may work on the code in the future.
- Test Your Validation Logic: Make sure to write tests that verify your validation rules work as expected.
Advanced Uses of Data Annotations
As your application scales, you may encounter cases where basic data annotations are not sufficient. Here are advanced strategies:
Custom Validation Attributes
When built-in attributes don't meet your needs, you can create custom validation attributes by inheriting from ValidationAttribute. This allows you to define complex validation scenarios tailored to your business logic.
public class MustBeAdultAttribute : ValidationAttribute { protected override ValidationResult IsValid(object value, ValidationContext validationContext) { int age = (int)value; if (age user.Name).NotEmpty().WithMessage("Name is required."); RuleFor(user => user.Email).EmailAddress().WithMessage("Invalid email address."); RuleFor(user => user.Age).InclusiveBetween(18, 100).WithMessage("Age must be between 18 and 100."); } }Conclusion: The Future of Data Annotations in Software Development
As software development evolves, the role of data annotations continues to expand. They not only enhance data integrity but also streamline development processes, paving the way for more robust applications. By adopting data annotations, developers can focus more on value-adding features rather than getting bogged down in validation code.
Embracing this methodology will not only improve the efficiency and reliability of your application but will also make a positive impact on user experience. As a part of your toolkit, data annotations are indispensable for any serious software development project, especially within the dynamic landscape of modern application frameworks.
Learn More About Data Annotations and Software Development
To take your understanding of data annotations to the next level, explore detailed documentation and resources available on leading software development platforms, such as Microsoft docs. Staying informed and continually enhancing your skills is key to thriving in the ever-evolving tech industry.